This chapter is taken from the book - Getting started with Java programming language (https://www.amazon.com/dp/1544614519/). You can download the code for the book from here: https://drive.google.com/file/d/0B1IwsLB5TOglZXYxWW9JMndUX3M/view
Chapter 1 - Hello World!
1-1 Introduction
Java is an object-oriented programming language that simplifies writing computer programs. Java was introduced to programmers in 1995 by Sun Microsystems, and is currently maintained by Oracle Corporation. Java programs are platform-independent, that is, the behavior of Java programs remains the same irrespective of the platform (which means the hardware and the operating system) on which you run them. This means that the sample Java programs that accompany this book will behave the same way if you run them on Windows, Ubuntu, Mac, or any other system.
In this chapter, we’ll first look at how to download and install the following software that you’ll need for writing and running Java programs:
> JDK (Java SE Development Kit) – provides the necessary tools to compile and run Java programs
> Eclipse IDE – an integrated development environment (IDE) that simplifies writing, compiling, debugging and running Java programs
Once we’ve setup the development environment, we’ll look at a simple Java program that prints ‘Hello World !’ message. We’ll wrap this chapter up by looking at what makes Java programs platform-independent, and how to import programs contained in java-book-samples ZIP file into Eclipse IDE.
1-2 Setting up the development environment
To setup the development environment, you need to do the following:
> Download and install JDK and Eclipse IDE
> Configure Eclipse IDE to use the installed JDK
Let’s first look at how to download and install JDK and Eclipse IDE.
Downloading and installing JDK and Eclipse IDE
You can download JDK from Oracle’s website by going to the following URL: http://www.oracle.com/technetwork/java/javase/downloads/index.html. As shown in figure 1-1, click the Download option under JDK. This will open the web page (refer figure 1-2) that shows JDK downloads available for different processor types (32- and 64-bit) and operating systems. Accept the license agreement and click the JDK that is applicable to your system. For instance, if you are using a 64-bit Windows system, download jdk-8u101-windows-x64.exe. You can also view the installation instructions specific to your system by clicking the Installation Instructions link (refer figure 1-1).
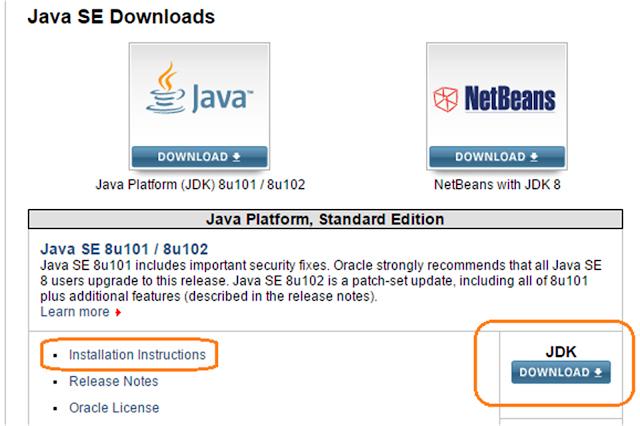
Figure 1-1 Click the Download option to go to the JDK download page
Figure 1-2 Accept the license agreement, and select the JDK applicable for your system
NOTE The name of JDK shown in figures 1-1 and 1-2 is 8u101, which means JDK’s version is 8 and its update number is 101. By default, Oracle’s website shows the latest JDK update. So, don’t be surprised if the JDK update number mentioned in this book doesn’t match with latest JDK available from Oracle’s website.
If you are using Windows, install JDK by double-clicking the downloaded .exe file and following the installation wizard. A JRE (Java Runtime Environment) is also installed along with JDK. By default, JDK is installed in C:\Program Files\Java\jdk1.8.0_101 folder, and JRE is installed in C:\Program Files\Java\jre1.8.0_101 folder. We’ll learn more about JDK and JRE later in this chapter.
To verify that JDK is successfully installed, open Windows command prompt and execute java -version command. The command prints the version of JDK installed, as shown here:
C:\>java -version
java version "1.8.0_101"
Java(TM) SE Runtime Environment (build 1.8.0_101-b13)
Java HotSpot(TM) Client VM (build 25.101-b13, mixed mode)
Figure 1-3 Choose the installer for your system and download
As shown in the above figure, choose the operating system and the processor type (32- or 64-bit) to download the Eclipse installer.
Figure 1-4 Select ‘Eclipse IDE for Java Developers’ from the list of options
If you select Windows operating system and 64-bit processor, you’ll get eclipse-inst-win64.exe file as the installer. To install Eclipse IDE, double-click the downloaded installer. As Eclipse IDE comes in many flavors and for many languages, choose ‘Eclipse IDE for Java Developers’ from the list of options (refer figure 1-4) that show up.
You’ll now be asked to specify the installation location of Eclipse IDE:
If the specified folder location doesn’t exist, the installer will automatically create it. We’ve chosen C:\myide folder as the location of installation. Click ‘INSTALL’ to start the installation. After Eclipse IDE is successfully installed, you’ll see the ‘LAUNCH’ button, as shown here:
Figure 1-6 ‘LAUNCH’ button is displayed after successful installation of Eclipse IDE
If you had chosen myide as the installation directory, Eclipse IDE is installed in C:\myide\eclipse folder. To start Eclipse IDE, click the ‘LAUNCH’ button or go to C:\myide\eclipse folder and double-click eclipse.exe file.
When you start Eclipse IDE, it asks for the workspace location – the location where Eclipse IDE saves Java programs. The following figure shows that we’ve set C:\java-samples folder as the workspace location:
Figure 1-7 Set the directory into which Java programs created via Eclipse IDE are saved
After you click OK button, Eclipse IDE’s welcome screen is shown:
Figure 1-8 Welcome screen is shown when you open Eclipse IDE
After you close the welcome screen, you’ll see different sections of Eclipse IDE (refer figure 1-9). You must familiarize yourself with Eclipse IDE, as you’ll be using it for writing and running programs. The Package Explorer tab on the left side of the IDE shows files and directories that form the Java programs. Any Java project or Java program that you create, will appear in the Package Explorer tab. The central area is the place where your currently open Java programs are displayed. This is the area where you’ll be editing your Java programs. The Problems tab at the bottom displays syntax errors that Eclipse IDE finds in your Java programs. As we work through Java programs in this book, we’ll look at different features offered by Eclipse IDE that simplify writing programs.
Figure 1-9 Important sections of Eclipse IDE – Package Explorer tab, Program editor area and Problems tab
Before you can use Eclipse IDE for writing Java programs, you need to tell Eclipse IDE about the installed JDK.
Configuring Eclipse IDE to use the installed JDK
Figure 1-10 Add the installed JDK to the list of installed JREs
To configure Eclipse IDE to use the JDK that we installed earlier, open Eclipse IDE preferences by selecting Windows -> Preferences option from the menu. As shown in figure 1-10, select Java -> Installed JREs option from the list of preferences.
Selecting the Installed JREs option displays the list of JREs that are currently configured with Eclipse IDE. The above figure shows that Eclipse IDE is already configured with the JRE located in C:\Program Files\Java\jre1.8.0_101 folder. To configure Eclipse IDE with JDK, click the Add button and select Standard JVM from the Add JRE dialog box:
Figure 1-11 Select Standard VM as the JRE Type
Click Next button to select the directory in which you had installed the JDK:
Figure 1-12 Select the JDK installation directory by clicking the Directory button
Once you have selected the JDK installation directory, click the Finish button.
The last step in configuring Eclipse IDE with JDK is to specify that the newly configured JDK must be used by default by Eclipse IDE:
Figure 1-13 Click OK to save changes in the preference
The above figure shows that jdk1.8.0_101 is checked, and jre1.8.0_101 is unchecked. Now, click OK to save changes in the preference.
You are now ready to create your first Java program.
1-3 Creating your first Java program
In this section, we’ll look at how to create a simple Java program that prints ‘Hello World !’ when we run it.
Before you create a Java program, you need to create a Java project in Eclipse IDE that will contain your Java program.
NOTE Java is a case-sensitive programming language; therefore, ensure that you are using the correct capitalization while creating the Java project and the Java program.
Creating a Java project
To create a Java project, go to Package Explorer tab and right-click inside it. This will show a popup menu, as shown in figure 1-14.
Figure 1-14 Select New -> Java Project to create a new Java project
Selecting New -> Java Project option opens the dialog box for creating a new Java project:
Figure 1-15 Enter project name and select Use default JRE option
Enter myproject as the name of the project, ensure that Use default location option is checked and Use default JRE option is selected. Click the Finish button to create myproject Java project. This will create a folder named myproject in the Project Explorer tab.
We are now ready to create Java programs in the newly created myproject.
Creating the ‘Hello World !’ program
Select myproject folder in the Package Explorer tab and right-click to view the popup menu. Select New -> Class, as shown here:
Figure 1-16 New -> Class is used to create a Java class where you write your program
New -> Class option shows the dialog box for creating a new Java class. A Java class is the file in which you write your Java program. The following figure shows the dialog box for creating a new Java class:
Figure 1-17 Enter HelloWorld as the name of the class and click Finish
The default value of Source folder and Package fields is myproject, which is the name of the Java project. Enter HelloWorld as the value of Name field and click Finish. This will create:
> a folder (referred to as a Java package) named myproject, and
> a file named HelloWorld.java inside myproject package
The following figure shows what is a Java project, package, class and program:
Figure 1-18 Java project, package, class and program in the context of myproject
HelloWorld.java file represents the HelloWorld Java class. Creating a Java class means you are creating a file with .java extension. You should note that Java programs are written inside .java files.
If you open the HelloWorld.java file by double-clicking it, you’ll see that it already contains the following text:
Listing 1-1 HelloWorld.java file
1 package myproject;
2
3 public class HelloWorld {
4
5 }
The above listing shows the bare minimum details that a Java class must contain. The actual HelloWorld.java file doesn’t have line numbers but we’ve specified them in the above listing. We’ve mentioned line numbers so that it is easy for us to discuss a particular line in a program. We’ll be following this practice throughout this book.
Let’s look at each of the lines in the HelloWorld.java file.
Line #1 – package myproject;
This line specifies that the HelloWorld class is inside the Java package named myproject. As shown in figure 1-18, HelloWorld.java file does reside inside myproject package.
Line #2 – blank lines have no significance in Java programs. They only help with readability of the program
Lines #3, #4 and #5 – defines the body of the HelloWorld class. You write your program logic inside opening '{' and closing '}' braces.
The .java file must contain the definition of the Java class in the following format:
public class <class-name>
here, <class-name> is the name of the Java class
The <class-name> must be same as the name of the .java file. For this reason, when we create HelloWorld class using Eclipse IDE, it creates a HelloWorld.java file and adds HelloWorld class definition inside it.
As we want to write a Java program that prints ‘Hello World !’, modify the HelloWorld.java file as shown here:
Listing 1-2 Modified HelloWorld.java file
1 package myproject;
2
3 public class HelloWorld {
4 public static void main(String[] args) {
5 System.out.println("Hello World !");
6 }
7 }
In the above listing, we’ve added a main method that prints ‘Hello World !’ message. A Java method contains a sequence of program instructions (enclosed within opening '{' and closing '}' braces) that are executed when that method is called. In the above listing, the main method contains only one program instruction System.out.println("Hello World !") that prints the ‘Hello World !’ message. After making changes to HelloWord.java file, save it by selecting File -> Save option from the menu bar or by using Ctrl + S key combination.
NOTE The contents of a .java file are referred to as source code or simply code.
To run the main method of HelloWorld class, do the following:
> open the HelloWorld.java file in the editor
> right-click inside the editor, and
> select Run As -> Java Application
Figure 1-19 shows that execution of the main method of HelloWorld class prints ‘Hello World !’ message in the Console tab.
NOTE A Java program is also referred to as a Java application. We’ll use the terms 'program' and 'application' interchangeably in this book.
Figure 1-19 Console tab shows the ‘Hello World !’ message printed by System.out.println
One of the advantages of using Eclipse IDE is that it’ll highlight portions of the code that don’t match the Java programming language rules. Figure 1-20 shows a scenario in which System.out.println was incorrectly typed as System.Out.println.
Figure 1-20 Errors in the Java class are highlighted by the Eclipse IDE editor and also displayed in the Problems tab
Figure 1-20 shows that the Eclipse IDE underlines Out part of System.Out.println in red and also shows an error message in the Problems tab to indicate that there is a problem with the HelloWorld class.
In this section, we created a HelloWorld class, myproject project and myproject package. Let’s look at how these Java artifacts are represented in the file system.
Java project structure
If you go to C:\java-samples directory (the workspace that we specified for use by Eclipse IDE), you’ll see the following directories and files:
Figure 1-21 The directory structure of myproject Java project
The above figure shows that a folder is created corresponding to both Java project and Java package. HelloWorld.java file is created corresponding to the HelloWorld class that we created via Eclipse IDE. HelloWorld.class file is created by Eclipse when it compiles the HelloWorld.java file. When you make changes to a .java file and save it, Eclipse IDE uses the Java compiler contained in the configured JDK to compile the .java file.
NOTE Java compiler is responsible for checking that the program instructions written in .java files are in accordance with the programming language rules. Java compiler is also responsible for converting a .java file into Java bytecode - a platform-independent format.
Let’s look at what makes Java a platform-independent programming language.
1-4 What makes Java platform-independent?
We saw in the previous section that Java programs are written in .java files. As shown in figure 1-22, program instructions contained in a .java file are converted into Java bytecode by the Java compiler. The Java compiler takes .java file as an input and creates a .class file that contains the bytecode. You can think of bytecode as an intermediate language between program instructions written in .java file and the machine language instructions understood by the underlying platform.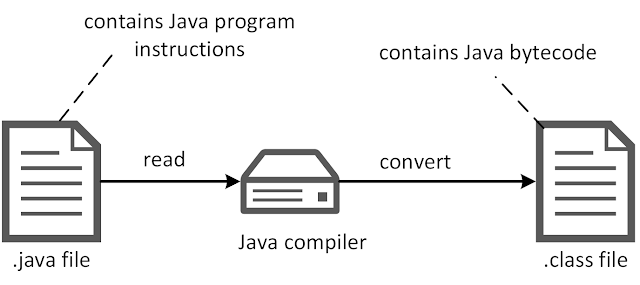
Figure 1-22 Java compiler converts program instructions written in .java file to Java bytecode
Java bytecode is platform-independent. This means, if you compile the HelloWorld.java file (refer listing 1-2) on Windows and on Linux, the generated .class files will be identical. The bytecode is understood by the Java Virtual Machine (JVM). JVM is part of Java Runtime Environment (JRE) that is responsible for running Java programs.
When you run a Java program, the following sequence of actions are performed by the JVM:
> reads the bytecode contained in .class file
> translates bytecode into machine language instructions understood by the underlying platform
> executes the translated machine language instructions
The following figure summarizes the role played by JVM in running Java programs: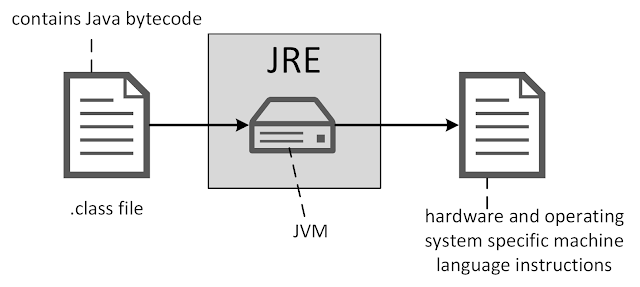
Figure 1-23 JVM converts bytecode into machine language instructions
As JVM is responsible for taking care of platform-specific requirements, you don’t need to worry about the platform on which you are writing the Java program or the platform on which you are running the compiled Java program. This is what makes Java a platform-independent programming language.
NOTE When you run a Java program using Run As -> Java Application option, the Eclipse IDE uses the JRE contained in the configured JDK to run the Java program.
JDK and JRE are at the heart of compiling and running Java programs. Let’s take a closer look at them.
JDK and JRE
We saw earlier that JDK was installed in C:\Program Files\Java\jdk1.8.0_101 folder. jdk1.8.0_101 folder’s bin directory contains executables that are used for compiling and running Java programs. The following table describes some of the executables contained in the installed JDK’s bin directory:
Table 1-1 – Development tools contained in JDK’s bin directory
NOTE When we save a .java file in Eclipse IDE, it internally uses javac to compile the .java file into a .class file. And, when we choose Run As -> Java Application option, Eclipse IDE uses java to run the class’s main method. The java executable in turn uses the JVM to execute the main method.
The jdk1.8.0_101 folder’s contains a jre folder that contains the Java Runtime Environment (JRE). The JRE contains the JVM (Java Virtual Machine) for translating the Java bytecode (contained in .class files) to the underlying platform and executing them.
We saw earlier that JRE is also installed in C:\Program Files\Java\jre1.8.0_101 folder. The JRE contained inside jdk1.8.0_101 folder is referred to as private JRE, and the JRE installed in jre1.8.0_101 folder is referred to as public JRE. The private JRE is only available to the JDK, and public JRE is meant to be used by other applications (like, web browser) installed in the system for running compiled Java programs.
NOTE As we configured Eclipse IDE to uses JDK, the Eclipse IDE uses the private JRE to run Java programs.
Let’s now look at how to import an existing project into Eclipse IDE.
1-5 Importing projects into Eclipse IDE
The java-book-code ZIP file that accompanies this book contains Eclipse IDE projects that you can directly import into your Eclipse IDE. If you unzip the java-book-code, you’ll see that it contains folders like myproject, chapter02, and so on. Each folder represents an Eclipse project that you can import into your Eclipse IDE.
To import a project, select File -> Import option in the Eclipse IDE:
Figure 1-24 Choose the File -> Import option to import one or more Eclipse projects into IDE.
As we want to import existing Eclipse IDE projects, select the ‘Existing Projects into Workspace’ option from the dialog box and click ‘Next >’ button:
Figure 1-25 Select ‘Existing Projects into Workspace’ option to import existing Eclipse projects into Eclipse IDE
You’ll now be asked to select the folder that contains one or more Eclipse projects:
Figure 1-26 Click the Browse button to choose the folder inside java-book-code that you want to import into Eclipse IDE.
Click the Browse button and select the project subfolder inside java-book-code that you want to import into your Eclipse IDE. For instance, if you want to import chapter02 project, then select the chapter02 folder of java-book-code. Once you have selected the project folder, click the Finish button to import the project into Eclipse IDE.
NOTE You can import all the projects inside java-book-code folder by selecting the java-book-code folder itself.
QUIZ1-1 Which of the following statements are true?
a) Java programs are written in files with .java extension
b) Eclipse IDE simplifies writing and running Java programs
c) You need JDK for ensuring that Java programs are correctly written
d) Java is a platform-dependent programming language
1-2 Which of the following statements are true?
a) JDK contains JRE, and JRE contains JVM
b) JDK has a private JRE that is not available to other programs
c) You can run Java programs without using JDK
d) JRE is used for compiling .java files to .class files
e) Java bytecode is not compatible across different platforms
f) Java bytecode and JVM provide Java’s platform-independence capability
g) Instead of using Eclipse IDE, you can directly use javac and java executables in JDK’s bin directory to compile and run Java programs
1-6 Summary
In this chapter, we looked at how to set up JDK and Eclipse IDE, and how to write and run a simple Java program that prints ‘Hello World !’. In the next chapter, we’ll look at the concept of variables, data types and operators in Java.
Chapter 1 - Hello World!
1-1 Introduction
Java is an object-oriented programming language that simplifies writing computer programs. Java was introduced to programmers in 1995 by Sun Microsystems, and is currently maintained by Oracle Corporation. Java programs are platform-independent, that is, the behavior of Java programs remains the same irrespective of the platform (which means the hardware and the operating system) on which you run them. This means that the sample Java programs that accompany this book will behave the same way if you run them on Windows, Ubuntu, Mac, or any other system.
In this chapter, we’ll first look at how to download and install the following software that you’ll need for writing and running Java programs:
> JDK (Java SE Development Kit) – provides the necessary tools to compile and run Java programs
> Eclipse IDE – an integrated development environment (IDE) that simplifies writing, compiling, debugging and running Java programs
Once we’ve setup the development environment, we’ll look at a simple Java program that prints ‘Hello World !’ message. We’ll wrap this chapter up by looking at what makes Java programs platform-independent, and how to import programs contained in java-book-samples ZIP file into Eclipse IDE.
1-2 Setting up the development environment
To setup the development environment, you need to do the following:
> Download and install JDK and Eclipse IDE
> Configure Eclipse IDE to use the installed JDK
Let’s first look at how to download and install JDK and Eclipse IDE.
Downloading and installing JDK and Eclipse IDE
You can download JDK from Oracle’s website by going to the following URL: http://www.oracle.com/technetwork/java/javase/downloads/index.html. As shown in figure 1-1, click the Download option under JDK. This will open the web page (refer figure 1-2) that shows JDK downloads available for different processor types (32- and 64-bit) and operating systems. Accept the license agreement and click the JDK that is applicable to your system. For instance, if you are using a 64-bit Windows system, download jdk-8u101-windows-x64.exe. You can also view the installation instructions specific to your system by clicking the Installation Instructions link (refer figure 1-1).
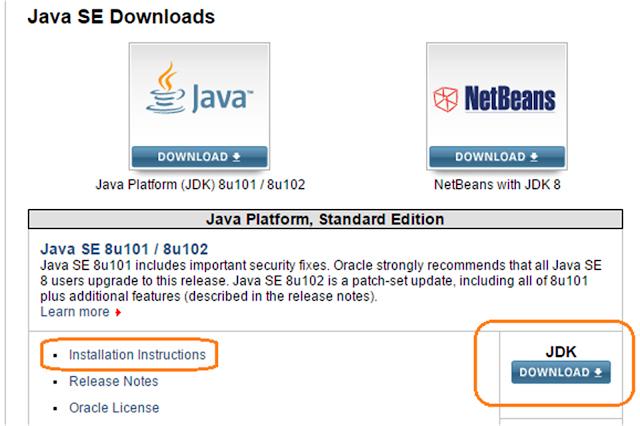
Figure 1-1 Click the Download option to go to the JDK download page
Figure 1-2 Accept the license agreement, and select the JDK applicable for your system
NOTE The name of JDK shown in figures 1-1 and 1-2 is 8u101, which means JDK’s version is 8 and its update number is 101. By default, Oracle’s website shows the latest JDK update. So, don’t be surprised if the JDK update number mentioned in this book doesn’t match with latest JDK available from Oracle’s website.
If you are using Windows, install JDK by double-clicking the downloaded .exe file and following the installation wizard. A JRE (Java Runtime Environment) is also installed along with JDK. By default, JDK is installed in C:\Program Files\Java\jdk1.8.0_101 folder, and JRE is installed in C:\Program Files\Java\jre1.8.0_101 folder. We’ll learn more about JDK and JRE later in this chapter.
To verify that JDK is successfully installed, open Windows command prompt and execute java -version command. The command prints the version of JDK installed, as shown here:
C:\>java -version
java version "1.8.0_101"
Java(TM) SE Runtime Environment (build 1.8.0_101-b13)
Java HotSpot(TM) Client VM (build 25.101-b13, mixed mode)
Once you have installed JDK, the next step is to download and install Eclipse IDE. You can download Eclipse IDE installer from the Eclipse website: http://www.eclipse.org/downloads/eclipse-packages/. Eclipse IDE Installers are available for different operating systems and processor types, as shown in the following figure:
Figure 1-3 Choose the installer for your system and download
As shown in the above figure, choose the operating system and the processor type (32- or 64-bit) to download the Eclipse installer.
If you select Windows operating system and 64-bit processor, you’ll get eclipse-inst-win64.exe file as the installer. To install Eclipse IDE, double-click the downloaded installer. As Eclipse IDE comes in many flavors and for many languages, choose ‘Eclipse IDE for Java Developers’ from the list of options (refer figure 1-4) that show up.
You’ll now be asked to specify the installation location of Eclipse IDE:
Figure 1-5 Choose the folder in which you want to install Eclipse IDE
If the specified folder location doesn’t exist, the installer will automatically create it. We’ve chosen C:\myide folder as the location of installation. Click ‘INSTALL’ to start the installation. After Eclipse IDE is successfully installed, you’ll see the ‘LAUNCH’ button, as shown here:
Figure 1-6 ‘LAUNCH’ button is displayed after successful installation of Eclipse IDE
If you had chosen myide as the installation directory, Eclipse IDE is installed in C:\myide\eclipse folder. To start Eclipse IDE, click the ‘LAUNCH’ button or go to C:\myide\eclipse folder and double-click eclipse.exe file.
When you start Eclipse IDE, it asks for the workspace location – the location where Eclipse IDE saves Java programs. The following figure shows that we’ve set C:\java-samples folder as the workspace location:
Figure 1-7 Set the directory into which Java programs created via Eclipse IDE are saved
After you click OK button, Eclipse IDE’s welcome screen is shown:
Figure 1-8 Welcome screen is shown when you open Eclipse IDE
After you close the welcome screen, you’ll see different sections of Eclipse IDE (refer figure 1-9). You must familiarize yourself with Eclipse IDE, as you’ll be using it for writing and running programs. The Package Explorer tab on the left side of the IDE shows files and directories that form the Java programs. Any Java project or Java program that you create, will appear in the Package Explorer tab. The central area is the place where your currently open Java programs are displayed. This is the area where you’ll be editing your Java programs. The Problems tab at the bottom displays syntax errors that Eclipse IDE finds in your Java programs. As we work through Java programs in this book, we’ll look at different features offered by Eclipse IDE that simplify writing programs.
Figure 1-9 Important sections of Eclipse IDE – Package Explorer tab, Program editor area and Problems tab
Before you can use Eclipse IDE for writing Java programs, you need to tell Eclipse IDE about the installed JDK.
Configuring Eclipse IDE to use the installed JDK
To configure Eclipse IDE to use the JDK that we installed earlier, open Eclipse IDE preferences by selecting Windows -> Preferences option from the menu. As shown in figure 1-10, select Java -> Installed JREs option from the list of preferences.
Selecting the Installed JREs option displays the list of JREs that are currently configured with Eclipse IDE. The above figure shows that Eclipse IDE is already configured with the JRE located in C:\Program Files\Java\jre1.8.0_101 folder. To configure Eclipse IDE with JDK, click the Add button and select Standard JVM from the Add JRE dialog box:
Figure 1-11 Select Standard VM as the JRE Type
Click Next button to select the directory in which you had installed the JDK:
Figure 1-12 Select the JDK installation directory by clicking the Directory button
Once you have selected the JDK installation directory, click the Finish button.
The last step in configuring Eclipse IDE with JDK is to specify that the newly configured JDK must be used by default by Eclipse IDE:
Figure 1-13 Click OK to save changes in the preference
The above figure shows that jdk1.8.0_101 is checked, and jre1.8.0_101 is unchecked. Now, click OK to save changes in the preference.
You are now ready to create your first Java program.
1-3 Creating your first Java program
In this section, we’ll look at how to create a simple Java program that prints ‘Hello World !’ when we run it.
Before you create a Java program, you need to create a Java project in Eclipse IDE that will contain your Java program.
NOTE Java is a case-sensitive programming language; therefore, ensure that you are using the correct capitalization while creating the Java project and the Java program.
Creating a Java project
To create a Java project, go to Package Explorer tab and right-click inside it. This will show a popup menu, as shown in figure 1-14.
Figure 1-14 Select New -> Java Project to create a new Java project
Selecting New -> Java Project option opens the dialog box for creating a new Java project:
Figure 1-15 Enter project name and select Use default JRE option
Enter myproject as the name of the project, ensure that Use default location option is checked and Use default JRE option is selected. Click the Finish button to create myproject Java project. This will create a folder named myproject in the Project Explorer tab.
We are now ready to create Java programs in the newly created myproject.
Creating the ‘Hello World !’ program
Select myproject folder in the Package Explorer tab and right-click to view the popup menu. Select New -> Class, as shown here:
Figure 1-16 New -> Class is used to create a Java class where you write your program
New -> Class option shows the dialog box for creating a new Java class. A Java class is the file in which you write your Java program. The following figure shows the dialog box for creating a new Java class:
Figure 1-17 Enter HelloWorld as the name of the class and click Finish
The default value of Source folder and Package fields is myproject, which is the name of the Java project. Enter HelloWorld as the value of Name field and click Finish. This will create:
> a folder (referred to as a Java package) named myproject, and
> a file named HelloWorld.java inside myproject package
The following figure shows what is a Java project, package, class and program:
Figure 1-18 Java project, package, class and program in the context of myproject
HelloWorld.java file represents the HelloWorld Java class. Creating a Java class means you are creating a file with .java extension. You should note that Java programs are written inside .java files.
If you open the HelloWorld.java file by double-clicking it, you’ll see that it already contains the following text:
Listing 1-1 HelloWorld.java file
1 package myproject;
2
3 public class HelloWorld {
4
5 }
The above listing shows the bare minimum details that a Java class must contain. The actual HelloWorld.java file doesn’t have line numbers but we’ve specified them in the above listing. We’ve mentioned line numbers so that it is easy for us to discuss a particular line in a program. We’ll be following this practice throughout this book.
Let’s look at each of the lines in the HelloWorld.java file.
Line #1 – package myproject;
This line specifies that the HelloWorld class is inside the Java package named myproject. As shown in figure 1-18, HelloWorld.java file does reside inside myproject package.
Line #2 – blank lines have no significance in Java programs. They only help with readability of the program
Lines #3, #4 and #5 – defines the body of the HelloWorld class. You write your program logic inside opening '{' and closing '}' braces.
The .java file must contain the definition of the Java class in the following format:
public class <class-name>
here, <class-name> is the name of the Java class
The <class-name> must be same as the name of the .java file. For this reason, when we create HelloWorld class using Eclipse IDE, it creates a HelloWorld.java file and adds HelloWorld class definition inside it.
As we want to write a Java program that prints ‘Hello World !’, modify the HelloWorld.java file as shown here:
Listing 1-2 Modified HelloWorld.java file
1 package myproject;
2
3 public class HelloWorld {
4 public static void main(String[] args) {
5 System.out.println("Hello World !");
6 }
7 }
In the above listing, we’ve added a main method that prints ‘Hello World !’ message. A Java method contains a sequence of program instructions (enclosed within opening '{' and closing '}' braces) that are executed when that method is called. In the above listing, the main method contains only one program instruction System.out.println("Hello World !") that prints the ‘Hello World !’ message. After making changes to HelloWord.java file, save it by selecting File -> Save option from the menu bar or by using Ctrl + S key combination.
NOTE The contents of a .java file are referred to as source code or simply code.
To run the main method of HelloWorld class, do the following:
> open the HelloWorld.java file in the editor
> right-click inside the editor, and
> select Run As -> Java Application
Figure 1-19 shows that execution of the main method of HelloWorld class prints ‘Hello World !’ message in the Console tab.
NOTE A Java program is also referred to as a Java application. We’ll use the terms 'program' and 'application' interchangeably in this book.
Figure 1-19 Console tab shows the ‘Hello World !’ message printed by System.out.println
One of the advantages of using Eclipse IDE is that it’ll highlight portions of the code that don’t match the Java programming language rules. Figure 1-20 shows a scenario in which System.out.println was incorrectly typed as System.Out.println.
Figure 1-20 Errors in the Java class are highlighted by the Eclipse IDE editor and also displayed in the Problems tab
Figure 1-20 shows that the Eclipse IDE underlines Out part of System.Out.println in red and also shows an error message in the Problems tab to indicate that there is a problem with the HelloWorld class.
In this section, we created a HelloWorld class, myproject project and myproject package. Let’s look at how these Java artifacts are represented in the file system.
Java project structure
If you go to C:\java-samples directory (the workspace that we specified for use by Eclipse IDE), you’ll see the following directories and files:
Figure 1-21 The directory structure of myproject Java project
The above figure shows that a folder is created corresponding to both Java project and Java package. HelloWorld.java file is created corresponding to the HelloWorld class that we created via Eclipse IDE. HelloWorld.class file is created by Eclipse when it compiles the HelloWorld.java file. When you make changes to a .java file and save it, Eclipse IDE uses the Java compiler contained in the configured JDK to compile the .java file.
NOTE Java compiler is responsible for checking that the program instructions written in .java files are in accordance with the programming language rules. Java compiler is also responsible for converting a .java file into Java bytecode - a platform-independent format.
Let’s look at what makes Java a platform-independent programming language.
1-4 What makes Java platform-independent?
We saw in the previous section that Java programs are written in .java files. As shown in figure 1-22, program instructions contained in a .java file are converted into Java bytecode by the Java compiler. The Java compiler takes .java file as an input and creates a .class file that contains the bytecode. You can think of bytecode as an intermediate language between program instructions written in .java file and the machine language instructions understood by the underlying platform.
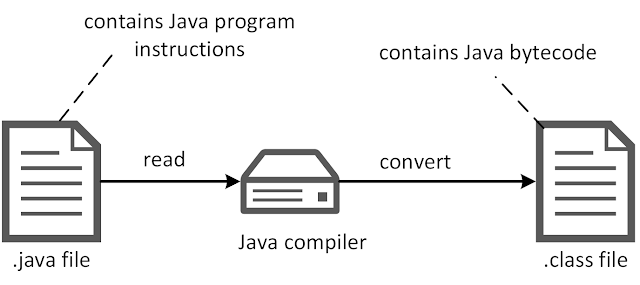
Figure 1-22 Java compiler converts program instructions written in .java file to Java bytecode
Java bytecode is platform-independent. This means, if you compile the HelloWorld.java file (refer listing 1-2) on Windows and on Linux, the generated .class files will be identical. The bytecode is understood by the Java Virtual Machine (JVM). JVM is part of Java Runtime Environment (JRE) that is responsible for running Java programs.
When you run a Java program, the following sequence of actions are performed by the JVM:
> reads the bytecode contained in .class file
> translates bytecode into machine language instructions understood by the underlying platform
> executes the translated machine language instructions
The following figure summarizes the role played by JVM in running Java programs:
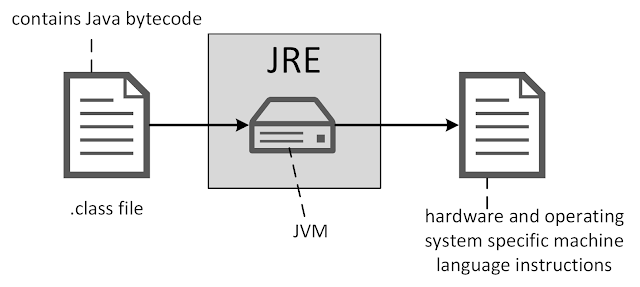
Figure 1-23 JVM converts bytecode into machine language instructions
As JVM is responsible for taking care of platform-specific requirements, you don’t need to worry about the platform on which you are writing the Java program or the platform on which you are running the compiled Java program. This is what makes Java a platform-independent programming language.
NOTE When you run a Java program using Run As -> Java Application option, the Eclipse IDE uses the JRE contained in the configured JDK to run the Java program.
JDK and JRE are at the heart of compiling and running Java programs. Let’s take a closer look at them.
JDK and JRE
We saw earlier that JDK was installed in C:\Program Files\Java\jdk1.8.0_101 folder. jdk1.8.0_101 folder’s bin directory contains executables that are used for compiling and running Java programs. The following table describes some of the executables contained in the installed JDK’s bin directory:
Table 1-1 – Development tools contained in JDK’s bin directory
The jdk1.8.0_101 folder’s contains a jre folder that contains the Java Runtime Environment (JRE). The JRE contains the JVM (Java Virtual Machine) for translating the Java bytecode (contained in .class files) to the underlying platform and executing them.
We saw earlier that JRE is also installed in C:\Program Files\Java\jre1.8.0_101 folder. The JRE contained inside jdk1.8.0_101 folder is referred to as private JRE, and the JRE installed in jre1.8.0_101 folder is referred to as public JRE. The private JRE is only available to the JDK, and public JRE is meant to be used by other applications (like, web browser) installed in the system for running compiled Java programs.
NOTE As we configured Eclipse IDE to uses JDK, the Eclipse IDE uses the private JRE to run Java programs.
Let’s now look at how to import an existing project into Eclipse IDE.
1-5 Importing projects into Eclipse IDE
The java-book-code ZIP file that accompanies this book contains Eclipse IDE projects that you can directly import into your Eclipse IDE. If you unzip the java-book-code, you’ll see that it contains folders like myproject, chapter02, and so on. Each folder represents an Eclipse project that you can import into your Eclipse IDE.
To import a project, select File -> Import option in the Eclipse IDE:
Figure 1-24 Choose the File -> Import option to import one or more Eclipse projects into IDE.
As we want to import existing Eclipse IDE projects, select the ‘Existing Projects into Workspace’ option from the dialog box and click ‘Next >’ button:
Figure 1-25 Select ‘Existing Projects into Workspace’ option to import existing Eclipse projects into Eclipse IDE
You’ll now be asked to select the folder that contains one or more Eclipse projects:
Figure 1-26 Click the Browse button to choose the folder inside java-book-code that you want to import into Eclipse IDE.
Click the Browse button and select the project subfolder inside java-book-code that you want to import into your Eclipse IDE. For instance, if you want to import chapter02 project, then select the chapter02 folder of java-book-code. Once you have selected the project folder, click the Finish button to import the project into Eclipse IDE.
NOTE You can import all the projects inside java-book-code folder by selecting the java-book-code folder itself.
QUIZ1-1 Which of the following statements are true?
a) Java programs are written in files with .java extension
b) Eclipse IDE simplifies writing and running Java programs
c) You need JDK for ensuring that Java programs are correctly written
d) Java is a platform-dependent programming language
1-2 Which of the following statements are true?
a) JDK contains JRE, and JRE contains JVM
b) JDK has a private JRE that is not available to other programs
c) You can run Java programs without using JDK
d) JRE is used for compiling .java files to .class files
e) Java bytecode is not compatible across different platforms
f) Java bytecode and JVM provide Java’s platform-independence capability
g) Instead of using Eclipse IDE, you can directly use javac and java executables in JDK’s bin directory to compile and run Java programs
1-6 Summary
In this chapter, we looked at how to set up JDK and Eclipse IDE, and how to write and run a simple Java program that prints ‘Hello World !’. In the next chapter, we’ll look at the concept of variables, data types and operators in Java.
Comments
Post a Comment